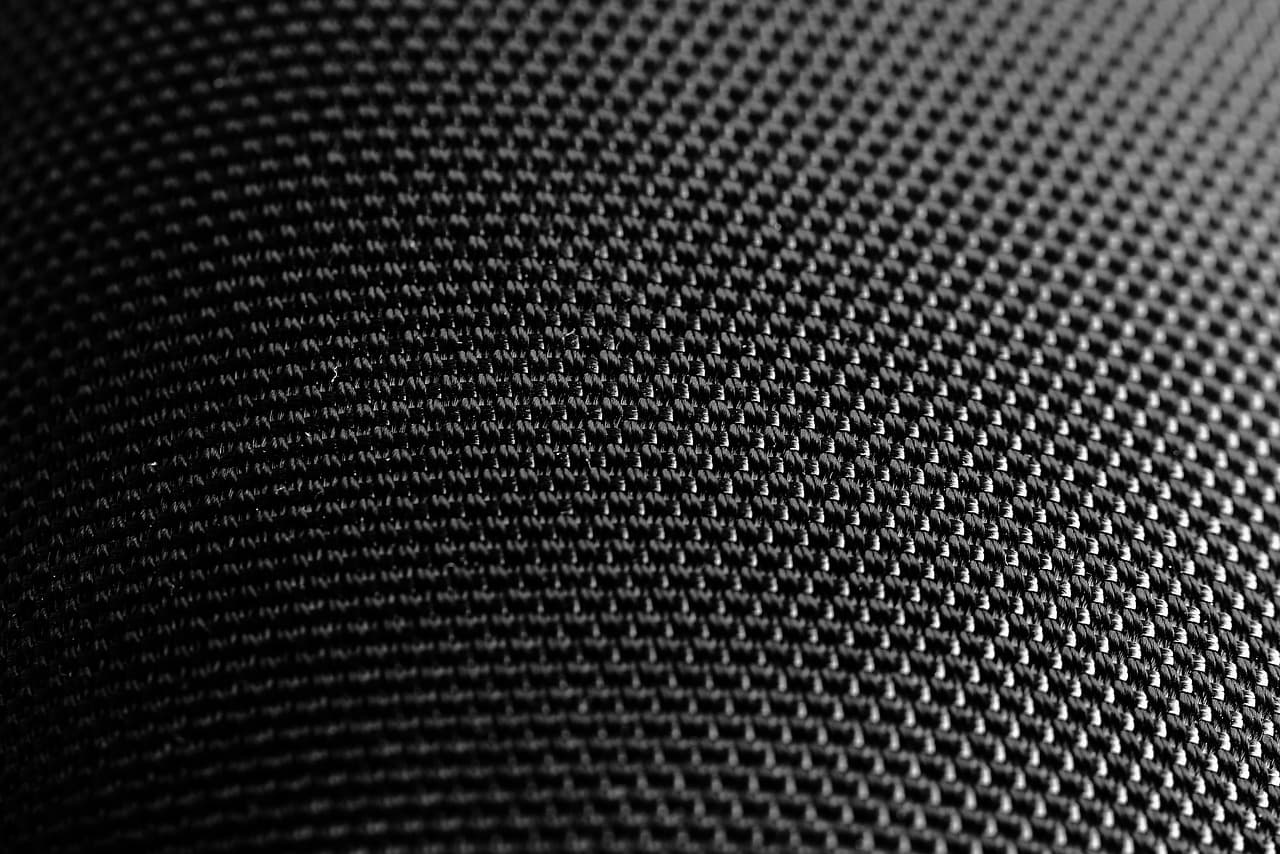
React: Understanding React Error Boundaries
React is a powerful library for building user interfaces, but like any software, it's not immune to errors. When an error occurs in a React component, it can potentially crash the entire application. This is where Error Boundaries come into play, providing a robust way to handle errors gracefully. In this blog post, we’ll explore what Error Boundaries are, how to implement them, and discuss some use cases.
What is an Error Boundary?
An Error Boundary is a special type of React component that catches JavaScript errors anywhere in its child component tree. It allows you to log errors and display a fallback UI instead of crashing the whole application. Error Boundaries work similarly to try/catch blocks in JavaScript but are specific to React components.
Key Points About Error Boundaries:
-
Only Class Components: Error boundaries can only be implemented in class components (as of React 16). Functional components can’t use error boundaries directly, but you can wrap them in class components.
-
Lifecycle Methods: Error boundaries use the
componentDidCatch(error, info)
lifecycle method to log error information and perform side effects. -
Fallback UI: You can define a fallback UI that displays when an error occurs.
How to Create an Error Boundary
Let’s create a simple Error Boundary component:
import React from 'react';
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
// Update state so the next render shows the fallback UI
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
// Log the error to an error reporting service
console.error("Error caught in Error Boundary:", error, errorInfo);
}
render() {
if (this.state.hasError) {
// Fallback UI
return <h1>Something went wrong. Please try again later.</h1>;
}
return this.props.children;
}
}
Usage Example
Now that we have an Error Boundary component, let’s use it in our application.
import React from 'react';
import ReactDOM from 'react-dom';
function BuggyComponent() {
// This will throw an error
throw new Error('I crashed!');
}
function App() {
return (
<div>
<h1>My Application</h1>
<ErrorBoundary>
<BuggyComponent />
</ErrorBoundary>
</div>
);
}
ReactDOM.render(<App />, document.getElementById('root'));
In this example, when BuggyComponent
throws an error, the Error Boundary will catch it and display the fallback UI instead of crashing the entire app.
When to Use Error Boundaries
-
Critical Components: Use Error Boundaries around components that are critical for the user experience. If they fail, it’s better to show a fallback than to break the entire app.
-
Third-Party Libraries: When integrating third-party libraries, wrapping their components in an Error Boundary can prevent their errors from propagating and crashing your application.
-
Dynamic Content: For components that display dynamic content or data fetched from APIs, wrapping them in an Error Boundary can handle unexpected issues gracefully.
Limitations of Error Boundaries
While Error Boundaries are powerful, they have some limitations:
-
Lifecycle Methods: They do not catch errors for event handlers, asynchronous code, server-side rendering, or errors thrown in the error boundary itself.
-
Not Global: You’ll need to wrap individual components or sections of your app. There’s no catch-all error boundary unless you implement it at the top level.
-
Performance: Overusing error boundaries can lead to performance issues if they cause unnecessary re-renders. Be judicious about where you apply them.
Conclusion
Error Boundaries are an essential part of building robust React applications. They provide a safety net for catching errors, allowing developers to create a smoother user experience by displaying fallback UIs instead of crashing the application. By implementing Error Boundaries wisely, you can enhance the resilience of your applications and improve overall user satisfaction.
As you build more complex applications, keep Error Boundaries in mind to handle errors gracefully and maintain a seamless user experience.