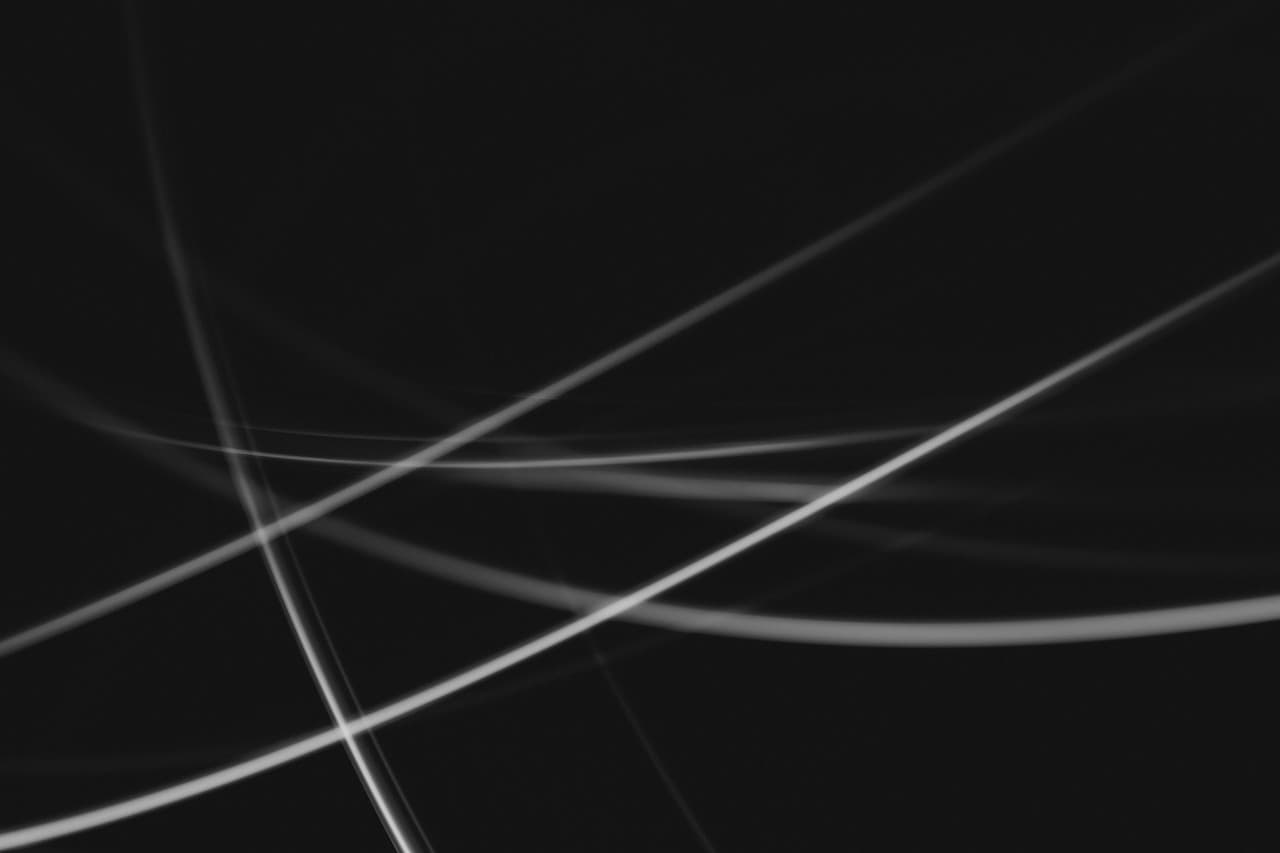
React: Thinking in React: A Guide to Building UIs
When it comes to building user interfaces (UIs) in web applications, React has established itself as a powerful library that enables developers to create dynamic and responsive applications. However, effectively using React requires a shift in how you think about building UIs. This approach, often referred to as "Thinking in React," encourages a component-based architecture that enhances maintainability, reusability, and scalability.
The Component-Based Architecture
At the core of React is the idea of components. These are reusable pieces of UI that encapsulate their own structure, styling, and behavior. Thinking in components helps break down a complex UI into smaller, manageable pieces. Here’s how to get started:
1. Break Down the UI into Components
Start by visualizing the UI and identifying its various parts. For instance, if you're building a simple e-commerce product page, consider the following components:
- Header: Contains navigation and branding.
- Product Image: Displays the product image.
- Product Details: Shows the name, description, and price.
- Add to Cart Button: Allows users to add the item to their shopping cart.
- Reviews Section: Displays user reviews and ratings.
By breaking down the UI into components, you create a clear structure that makes the application easier to manage.
2. Build a Static Version First
Before diving into interactivity, create a static version of your application. This means building your components without worrying about state or user interactions. Focus on the layout and rendering of your components, ensuring they display correctly.
For example, render the Product Image, Product Details, and Reviews Section with hardcoded data. This step allows you to visualize the entire UI and helps you identify potential areas for improvement.
3. Identify the State
Once you have a static version of your UI, identify which parts of your application need to maintain state. State refers to data that can change over time and affect how the UI renders. In our e-commerce example, the following elements may require state:
- The product's availability (in stock or out of stock).
- The selected image (if there are multiple images).
- The number of items in the cart.
Understanding state management is crucial, as it determines how components interact and update in response to user actions.
4. Determine How Components Will Interact
Think about how your components will communicate with each other. React promotes a unidirectional data flow, meaning that data flows from parent components to child components. This hierarchy helps manage complexity by ensuring that data is passed down as props.
For instance, the Add to Cart Button component might need to notify the parent component (e.g., the Product Details component) when an item is added to the cart. This interaction can be achieved by passing a function from the parent to the child as a prop.
5. Write the Logic for Updating State
After determining which components need to manage state and how they interact, you can implement the logic for updating state. This often involves defining functions that modify state and passing them as props to child components.
Using the e-commerce example, the Add to Cart Button can call a function passed from the parent when clicked. This function updates the cart's state, and React automatically re-renders the relevant components.
6. Optimize Performance
As your application grows, it's essential to keep performance in mind. React provides several tools and techniques for optimizing performance, such as:
- Memoization: Use
React.memo
to prevent unnecessary re-renders of functional components. - Code Splitting: Implement lazy loading with
React.lazy
andSuspense
to load components only when needed. - Avoiding Inline Functions: Define functions outside of the render method to prevent creating new instances on every render.
Conclusion
Thinking in React requires a paradigm shift in how you approach UI development. By focusing on a component-based architecture, breaking down your UI into manageable pieces, and effectively managing state, you can create applications that are not only functional but also maintainable and scalable.
As you practice and refine your skills, you'll find that this mindset fosters a deeper understanding of React and helps you build better user experiences. Embrace the power of components, and you’ll be well on your way to mastering React!