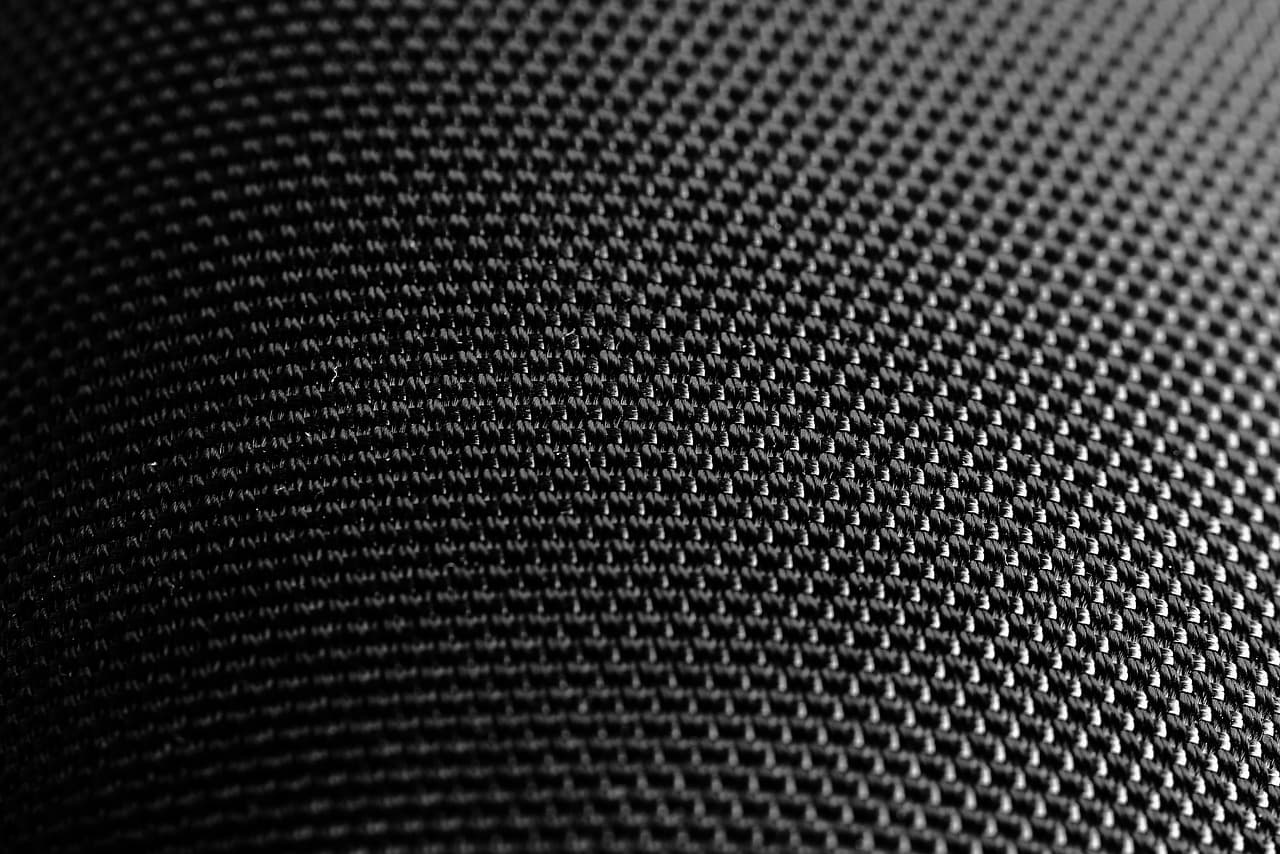
The Event Loop in Node.js: A Structured Guide for Kids (in depth)
Hey there, little coder! Are you ready to learn about something super cool that makes Node.js work like magic? It’s called the Event Loop! In this guide, we’ll start with the basics and go step by step, all the way to some advanced concepts. Let’s go!
Table of Contents
- What is Node.js?
- What is the Event Loop?
- Synchronous vs Asynchronous Code
- How the Code Executes: Call Stack, Message Queue, and Heap
- The Event Loop Phases
- How Libuv Helps the Event Loop
- Task Priority in the Event Loop
- Conclusion
What is Node.js?
Node.js is a special tool that helps you run JavaScript outside the browser. Normally, JavaScript is used to make web pages interactive, like when you click a button on a website. But with Node.js, you can use JavaScript to make server-side applications, like chat servers, web APIs, and more!
What is the Event Loop?
The Event Loop is like a super-smart robot inside Node.js that helps it handle many tasks at the same time. It makes sure things happen in the right order, even when some tasks take longer than others. This is important because computers need to do many things at once, like reading files, sending emails, and more.
Synchronous vs Asynchronous Code
Before we dive into the Event Loop, let’s understand two important types of code:
Synchronous Code
In synchronous code, tasks are done one at a time, in a straight line.
Example:
console.log('Task 1');
console.log('Task 2');
console.log('Task 3');
Here’s what happens:
- Task 1 happens first.
- Task 2 happens next.
- Task 3 happens last.
Each task has to finish before the next one can start.
Asynchronous Code
In asynchronous code, tasks can run at the same time! So while one task is waiting, the program can do other things.
Example:
console.log('Task 1');
setTimeout(() => {
console.log('Task 2');
}, 2000);
console.log('Task 3');
Here’s what happens:
- Task 1 happens first.
- The program sees that Task 2 is delayed for 2 seconds, so it moves on to Task 3.
- After 2 seconds, Task 2 gets printed.
This allows the program to do more than one thing at a time!
How the Code Executes: Call Stack, Message Queue, and Heap
When your program runs, the computer uses three important tools to handle tasks:
Call Stack
The Call Stack is like a pile of plates. Every time a new task starts, the computer places it on top of the pile. Once the task is done, the computer takes it off the stack.
Message Queue
The Message Queue is like a waiting line. If a task is asynchronous, it goes into the message queue to wait its turn to be executed after the stack is empty.
Heap
The Heap is like a storage area where data is kept while it’s being used by the program. It’s where we keep things like variables and objects.
The Event Loop Phases
The Event Loop is responsible for executing the code, handling asynchronous operations, and ensuring tasks are executed in the correct order. The Event Loop operates through phases that determine how different types of tasks (synchronous and asynchronous) are handled.
Here’s how it works:
The Phases of the Event Loop
The Event Loop works in phases to manage tasks in an orderly manner. Each phase has a specific role in processing different kinds of operations:
-
Timers: This phase executes tasks scheduled with
setTimeout()
andsetInterval()
. It runs after the specified delay. -
I/O Callbacks: Handles most of the operations related to I/O (e.g., reading files, network requests, interacting with databases).
-
Idle, Prepare: This phase is used internally by Node.js to prepare for the next loop cycle.
-
Poll: The Poll phase is where the Event Loop waits for I/O events to complete. It checks the message queue for new tasks and processes them. If no I/O events are pending, the Event Loop will move to the next phase.
-
Check: Executes
setImmediate()
callbacks. These are tasks that should run after the Poll phase, but before the next Event Loop cycle begins. -
Close Callbacks: This phase handles tasks like closing connections or cleaning up resources.
Microtask Queue and process.nextTick()
Apart from the main Event Loop phases, there are two important queues that the Event Loop processes before moving to the next phase:
-
Microtask Queue: This is a special queue for promises and other microtasks. Microtasks are executed after the currently executing script finishes, but before the Event Loop moves to the next phase. Microtasks have a higher priority than tasks in the Timers, Poll, and Check phases.
-
process.nextTick(): This is a special function that queues a task to run immediately after the current operation completes, but before the Event Loop continues.
process.nextTick()
callbacks are executed before any microtasks, which means they have the highest priority.
Example with Multiple Phases
Let’s look at an example of how the Event Loop phases interact:
console.log('Start');
setTimeout(() => {
console.log('Timeout');
}, 0);
setImmediate(() => {
console.log('Immediate');
});
process.nextTick(() => {
console.log('NextTick');
});
console.log('End');
Output:
Start
End
NextTick
Timeout
Immediate
- The code starts executing, printing Start and End.
- Then,
process.nextTick()
runs its callback immediately, printing NextTick. - Even though
setTimeout()
andsetImmediate()
are scheduled with 0 ms delay, NextTick is given priority and executes first. - After that, Timeout executes (from the Timers phase).
- Finally, Immediate executes (from the Check phase).
This shows how the Event Loop gives priority to process.nextTick()
over other asynchronous tasks.
How Libuv Helps the Event Loop
Libuv is a special library that Node.js uses to manage the Event Loop. It helps make sure that things run efficiently, even when tasks are waiting. Libuv takes care of the thread pool (a group of workers) and helps the Event Loop decide what to do next.
How Libuv Manages Async Tasks
Libuv divides its work into threads and event loops:
-
Main Thread: The main event loop runs in a single thread. It handles things like JavaScript execution,
setTimeout()
, and other simple tasks. -
Thread Pool: For operations that take time (e.g., file system access, DNS resolution, etc.), Libuv uses a thread pool to offload these operations so they don’t block the main thread. For example, reading a large file or querying a database might take time, so these tasks are delegated to separate threads, and the Event Loop continues processing other tasks in the meantime.
Thread Pool and Task Offloading
When you perform tasks like reading a file or interacting with a database, Node.js can offload these tasks to Libuv's thread pool, allowing the main thread (the Event Loop) to continue handling other tasks without waiting.
For example, when you use fs.readFile()
, Node.js doesn’t wait for the file to be read. Instead, it offloads this task to Libuv’s thread pool and moves on to other tasks. Once the file is read, Libuv notifies the Event Loop, which picks up the result and proceeds.
Task Priority in the Event Loop
Not all tasks are equal! Some tasks need to happen before others. Here’s a quick breakdown of task priority:
-
process.nextTick(): This has the highest priority and runs first.
-
Microtasks (Promises): These are also high priority and run after the current task finishes but before anything else.
-
Timers (setTimeout): These tasks are handled after microtasks.
-
I/O and Networking: These tasks can be handled at any point, depending on when the Event Loop is ready.
-
Close Events: These tasks happen last when everything else is finished.